Please Solve Part B only
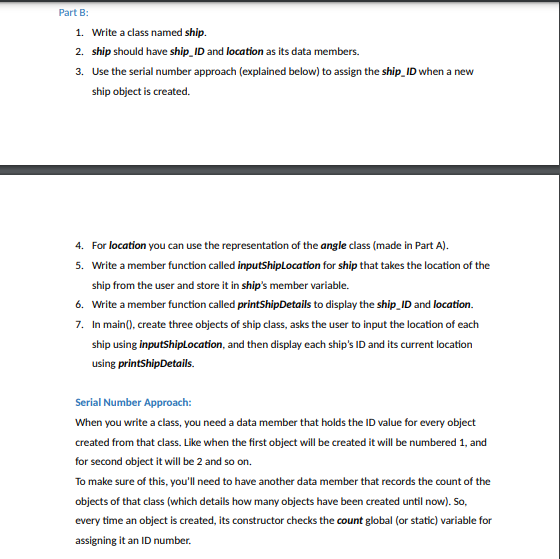
Extracted text: Part B: 1. Write a class named ship. 2. ship should have ship_ID and location as its data members. 3. Use the serial number approach (explained below) to assign the ship_ID when a new ship object is created. 4. For location you can use the representation of the angle class (made in Part A). 5. Write a member function called inputShipLocation for ship that takes the location of the ship from the user and store it in ship's member variable. 6. Write a member function called printShipDetails to display the ship_ID and location. 7. In main(), create three objects of ship class, asks the user to input the location of each ship using inputShiplocation, and then display each ship's ID and its current location using printShipDetails. Serial Number Approach: When you write a class, you need a data member that holds the ID value for every object created from that class. Like when the first object will be created it will be numbered 1, and for second object it will be 2 and so on. To make sure of this, you'll need to have another data member that records the count of the objects of that class (which details how many objects have been created until now). So, every time an object is created, its constructor checks the count global (or static) variable for assigning it an ID number.
![Part B isn't necessarily an extension of Part A; you're meant to do both of them separately, so<br>just write the exact same angle class for Part B (in step 4).<br>Part A:<br>1. Suppose you are sailing towards Island XYZ, your exact location facing towards XYZ is<br>158° 45.9' W, 28°42.6' S 1, The marine navigation system is measured in degrees and<br>minutes of longitude and latitude.<br>2. Write a class name angle, that have three data members named degrees, minutes and<br>direction. The data type for each member is int. float and char respectively.<br>3. angle can have either latitude or longitude variable, since only one of these is sufficient<br>to describe a location in the marine navigation system.<br>4. Write a parametrized constructor of class angle for all data members.<br>5. angle should have a member function called inputlocation that takes angle value (in<br>degrees and minutes) and direction from user.<br>Make sure of the following input constraints:<br>i. Direction we have these four char N, W, E and S.<br>ii. In a degree we have 60 minutes.<br>i. To measure latitude we have O to 90 degrees.<br>iv. To measure longitude we have O to 180 degrees.<br>6. Write a member function called printLocation to display the input values in the following<br>format: degrees ° minutes' direction<br>Example: 124° 12.7' E<br>7. Write a main() that display the values of an angle object initialized using inputlocation.<br>8. Use a sentinel control loop, that stops when the user enters 9992, otherwise it will<br>continue to take in angle values and display them as aforementioned.<br>[1] ° for degrees, 'for minutes, W for west longitude, and S for south latitude<br>Part B:<br>1. Write a class named ship.<br>](https://s3.us-east-1.amazonaws.com/storage.unifolks.com/qimg-008/008_m99tzz0-wgjkwnh2.png)
Extracted text: Part B isn't necessarily an extension of Part A; you're meant to do both of them separately, so just write the exact same angle class for Part B (in step 4). Part A: 1. Suppose you are sailing towards Island XYZ, your exact location facing towards XYZ is 158° 45.9' W, 28°42.6' S 1, The marine navigation system is measured in degrees and minutes of longitude and latitude. 2. Write a class name angle, that have three data members named degrees, minutes and direction. The data type for each member is int. float and char respectively. 3. angle can have either latitude or longitude variable, since only one of these is sufficient to describe a location in the marine navigation system. 4. Write a parametrized constructor of class angle for all data members. 5. angle should have a member function called inputlocation that takes angle value (in degrees and minutes) and direction from user. Make sure of the following input constraints: i. Direction we have these four char N, W, E and S. ii. In a degree we have 60 minutes. i. To measure latitude we have O to 90 degrees. iv. To measure longitude we have O to 180 degrees. 6. Write a member function called printLocation to display the input values in the following format: degrees ° minutes' direction Example: 124° 12.7' E 7. Write a main() that display the values of an angle object initialized using inputlocation. 8. Use a sentinel control loop, that stops when the user enters 9992, otherwise it will continue to take in angle values and display them as aforementioned. [1] ° for degrees, 'for minutes, W for west longitude, and S for south latitude Part B: 1. Write a class named ship.