Java. Refer to attachment. Starter code below.
public class Car
{
//attributes
private String make;
private String model;
private int year;
protected int speed;
//constructor
public Car(String make, String model, int year)
{
this.make = make;
this.model = model;
this.speed = 0;
this.year = year;
}
//Getters
public String getMake() {return make;}
public String getModel() {return model;}
public int getSpeed() {return speed;}
public int getYear() {return year;}
//Setters
public void setMake(String colour) {this.make = make;}
public void setModel(String colour){this.model = model;}
public void setSpeed(int speed) {this.speed = speed;}
public void setYear(int year) {this.year = year;}
public void accelerate() {
speed ++;
}
public void brake() {
speed --;
if (speed < 0)="" {speed="">
}
public String toString() {
String carDetails = "Make: " + this.make
+ "\nModel: " + this.model
+ "\nYear: " + this.year
+ "\nSpeed: " + this.speed;
return carDetails;
}
}
public class NissanLeaf extends Car {
//constructor
//override accelerate
//override brake
}
import java.util.Scanner;
public class PoD
{
public static void main (String [] arg )
{
Scanner in = new Scanner( System.in );
int num = in.nextInt();
String carType = in.next();
int carYear = in.nextInt();
Car newCar;
if (carType.equals("NissanLeaf"))
{
newCar = new NissanLeaf(carYear);
}
else
{
String make = carType;
String model = in.next();
newCar = new Car(make,model,carYear);
}
for (int i=0; i< num;="" i++)="">
for (int i=0; i< num/2;="" i++)="">
System.out.println(newCar);
in.close();
}
}
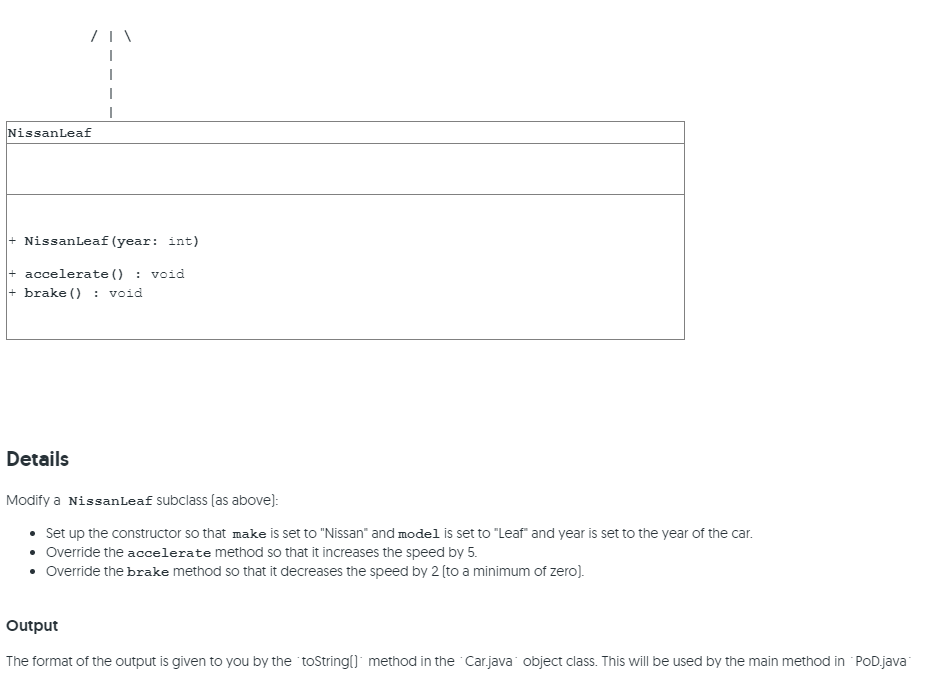
Extracted text: NissanLeaf + NissanLeaf(year: int) + accelerate () : void + brake () : void Details Modify a NissanLeaf subclass (as above): • Set up the constructor so that make is set to "Nissan" and model is set to "Leaf" and year is set to the year of the car. • Override the accelerate method so that it increases the speed by 5. • Override the brake method so that it decreases the speed by 2 (to a minimum of zero). Output The format of the output is given to you by the toString() method in the Car.java object class. This will be used by the main method in POD.java
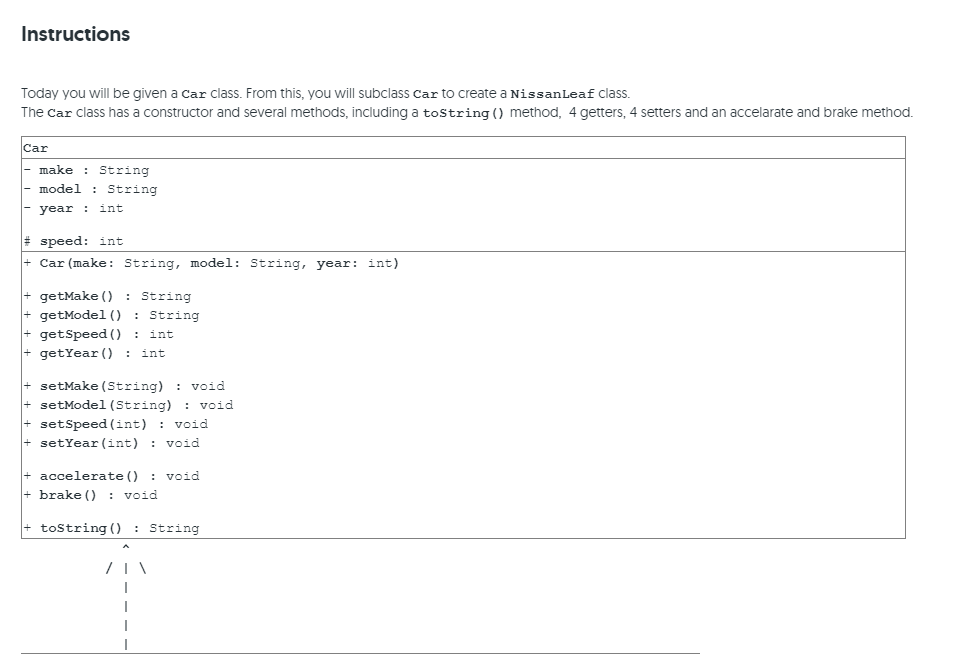
Extracted text: Instructions Today you willI be given a Car Class. From this, you will subclass Car to create a NissanLeaf Class. The Car class has a constructor and several methods, including a tostring () method, 4 getters, 4 setters and an accelarate and brake method. Car make : String model : String year : int # speed: int Car (make: String, model: String, year: int) getMake () : String getModel () : String + getSpeed () : int getYear () : int setMake (String) : void + setMode1 (String) : void setSpeed (int) : void + setYear (int) : void accelerate () : void brake () : void tostring () : String