In this assignment, you will design the AddNode and AddEdge methods for the supplied graph data structure. The AddNode and AddEdge methods are to support the construction of undirected (bi-directional) graphs. That is if node A is connected to node B then node B is also connected to node A.
In addition to the AddNode and AddEdge methods, create a method called BreadthFirstSearch that accepts a starting node and performs a Breadth First Search of the graph. The algorithm for the breadth first traversal is provided below
1. Add a node to the queue (starting node)
2. While the queue is not empty, dequeue a node
3. Add all unvisited nodes of the dequeued node from step 2 and add them to queue
4. End While
Demonstrate your methods by creating the graph depicted in Figure 1 below and running the Breadth First Search on the graph using 0 as the starting node.
(see image below)
You may use C++, C#, to implement this program as long as the following requirements are met. A C++, or C# project must be created using Visual Studio 2019.
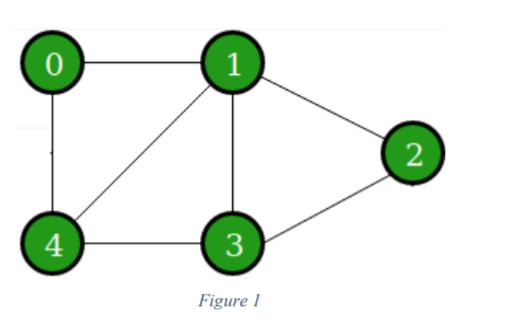
Extracted text: 1 2 4 3 Figure 1