In javaMake a program with classes named Vehicle, LandVehicle, AirVehicle, Car, Truck, Glider, Balloon, VehicleFactoryMain, MyFileManager.
Put all files in a package named "vehicleActivity"
The Vehicle Inheritance looks like this:
Note: Vehicle should also have a range.
(1st img here)
Make sure to override the toString() method for returning a String that includes all the information about an instance of a vehicle.
VehicleFactoryMain will use these classes to make a random number and assortment of vehicle types.
For example:
Car - red, 526Km,1524Kg, 1988Kg, 1001Kg, gas, 4-wheels, 2-doors, fastback.
Balloon - orange, 234Km,320Kg, 423Kg, 256Kg, gas, 950m, hot air.
For the various ranges, altitudes, and weights set a max and min value and randomly choose between those.
For values that have types (hot-air, hydrogen, helium or gas, electric, diesel, kerosene, methane,none) make a static array in the class and randomly choose one of the values.
Have the factory select a random number between 2-5 inclusive for each type of vehicle.
It will then generate that many randomized versions of one of the types of vehicles.
Repeat this process for all 4 types of vehicles and store all of them in an ArrayList.
-----------------------------------------------------------------------------------------------------------------------------------------
The MyFileManager class will have three methods:
clearFile(String fileName)
When this method is called, if the file with fileName exists, this method erases all text in the file.
appendLine(String lineToPrint, String fileName)
appendArray(ArrayList v, String fileName)
Anytime one of these methods is called, the file manager will check to see if the file exists. If it does, the String or array is appended to the list.
If the file does not exist, the MyFileManager creates it and writes to it.
All paths use just the file name so that the system will store and read/write them from the default path.
--------------------------------------------------------------------------------------------------------------------------------------------
After generating an array full of random vehicles, use your MyFileManager class to make a file named "VehicleProductionInventory.txt"
The text file will contain a header with the number of vehicles produced and the current date and time (ISO 8601 format).
Use metric measurements for all values
(2nd img)
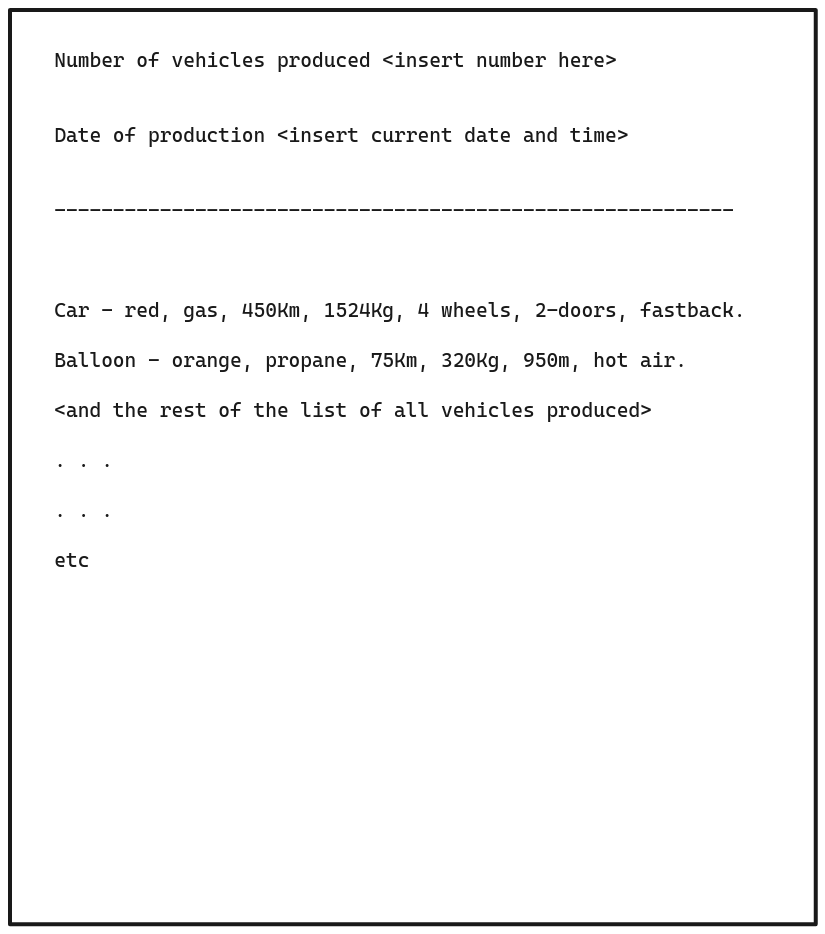
Extracted text: Number of vehicles produced Date of production Car red, gas, 450Km, 1524Kg, 4 wheels, 2-doors, fastback. Balloon - orange, propane, 75Km, 320Kg, 950m, hot air. etc
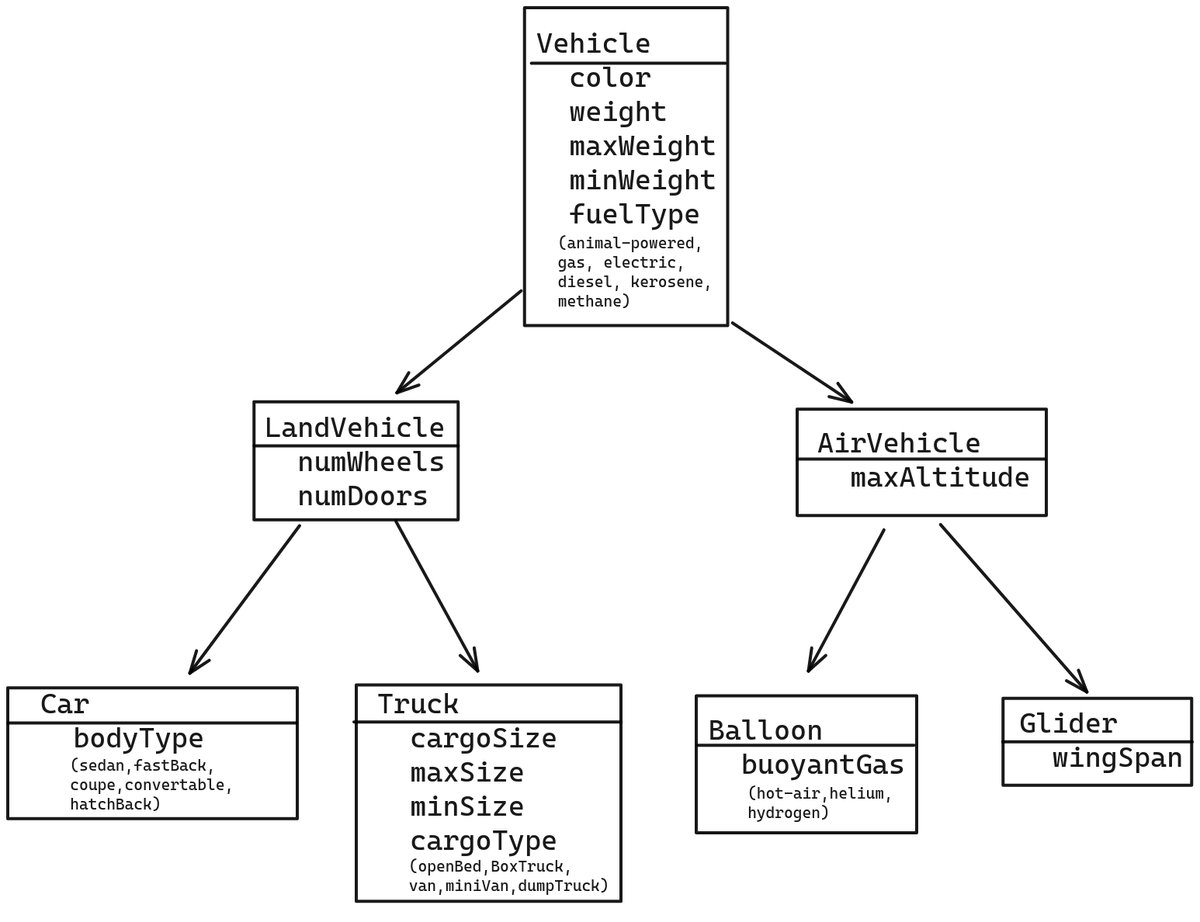
Extracted text: Vehicle color weight maxWeight minWeight fuelType (animal-powered, gas, electric, diesel, kerosene, methane) LandVehicle AirVehicle numWheels maxAltitude numDoors Car Truck Glider cargoSize Balloon buoyantGas bodyType wingSpan (sedan, fastBack, coupe, convertable, hatchBack) maxSize minSize (hot-air,helium, hydrogen) cargoType (openBed, BoxTruck, van, minivan, dumpTruck)