import
edu.princeton.cs.algs4.StdOut;
public
class
LinkedIntListR {
// helper linked list node class
private
class
Node {
private
int
item;
private
Node next;
public
Node(int
item, Node next) {
this.item = item;
this.next = next;
}
}
private
Node first; // first node of the list
public
LinkedIntListR() {
first =
null;
}
public
LinkedIntListR(int[] data) {
for
(int
i = data.length - 1; i >= 0; i--)
first =
new
Node(data[i], first);
}
public
void
addFirst(int
data) {
first =
new
Node(data, first);
}
public
void
printList() {
if
(first ==
null) {
StdOut.println("[]");
}
else
if
(first.next ==
null) {
StdOut.println("[" + first.item + "]");
}
else
{
StdOut.print("[" + first.item);
for(Node ptr = first.next; ptr !=
null; ptr = ptr.next)
StdOut.print(", " + ptr.item);
StdOut.println("]");
}
}
@Override
public
boolean
equals(Object obj) {
if
(this
== obj)
return
true;
if
(obj ==
null)
return
false;
if
(getClass() != obj.getClass())
return
false;
LinkedIntListR other = (LinkedIntListR) obj;
Node thisPtr = first;
Node otherPtr = other.first;
while(thisPtr !=
null
&& otherPtr !=
null) {
if
(thisPtr.item != otherPtr.item)
return
false;
thisPtr = thisPtr.next;
otherPtr = otherPtr.next;
}
if
(thisPtr !=
null
|| otherPtr !=
null)
return
false;
return
true;
}
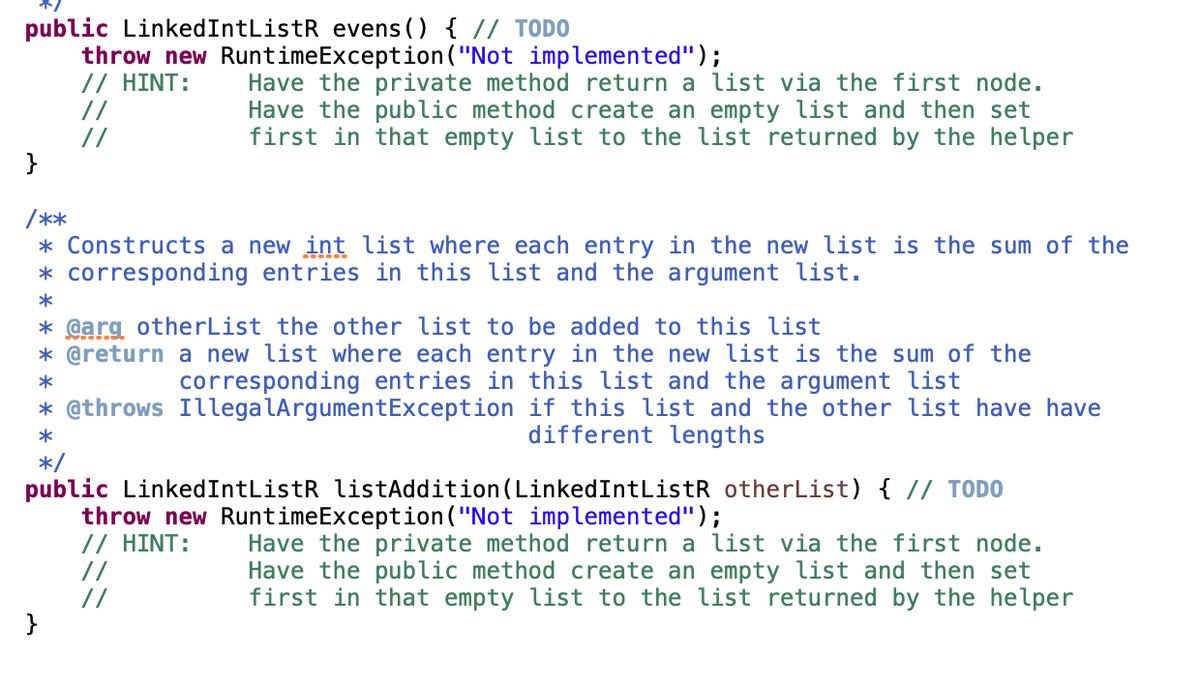
Extracted text: public LinkedIntListR evens () { // TODO throw new RuntimeException ("Not implemented"); // HINT: // // } Have the private method return a list via the first node. Have the public method create an empty list and then set first in that empty list to the list returned by the helper /** * Constructs a new int list where each entry in the new list is the sum of the * corresponding entries in this list and the argument list. * carg otherList the other list to be added to this list * @return a new list where each entry in the new list is the sum of the corresponding entries in this list and the argument list * @throws IllegalArgumentException if this list and the other list have have * different lengths */ public LinkedIntListR listAddition (LinkedIntListR otherList) { // TODO throw new RuntimeException("Not implemented"); // HINT: // // } Have the private method return a list via the first node. Have the public method create an empty list and then set first in that empty list to the list returned by the helper