Java. Please use the template, thank you!
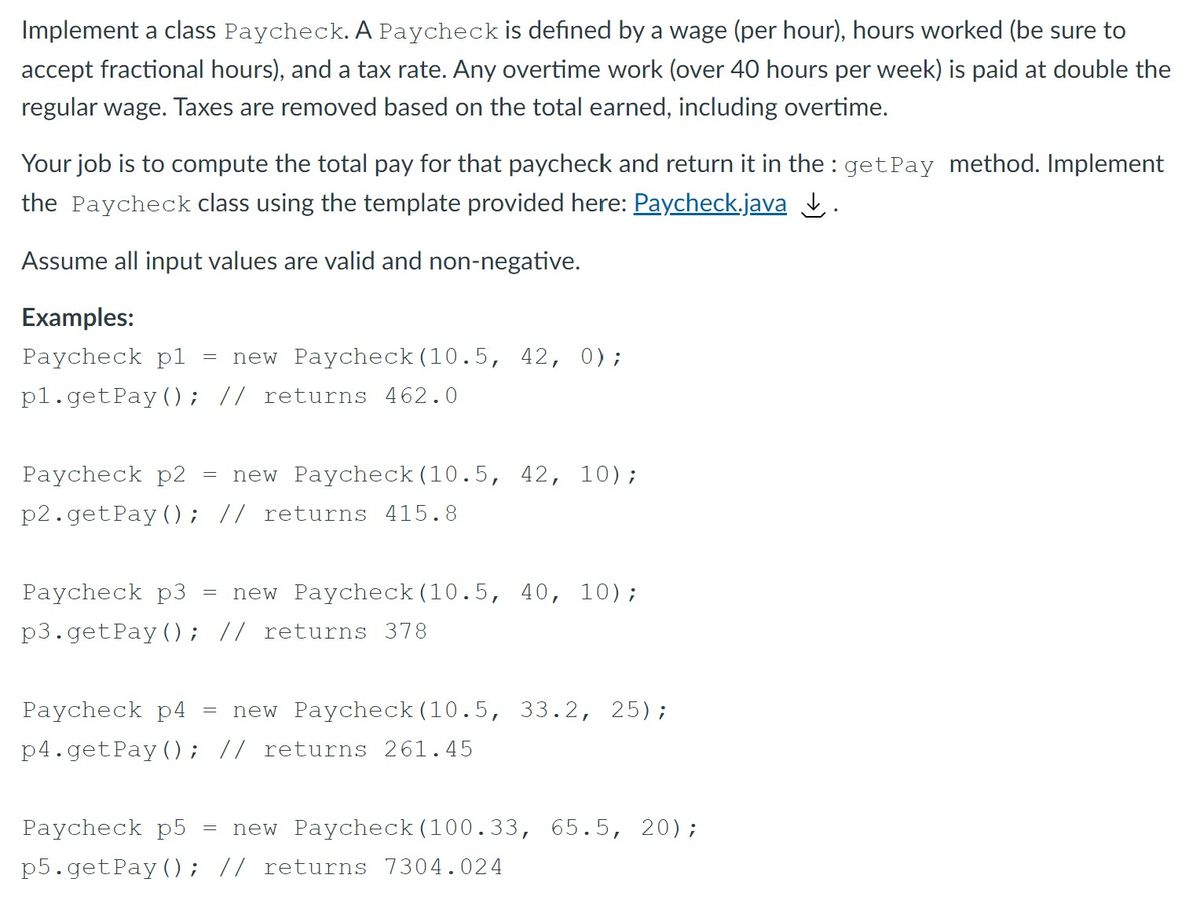
Extracted text: Implement a class Paycheck. A Paycheck is defined by a wage (per hour), hours worked (be sure to accept fractional hours), and a tax rate. Any overtime work (over 40 hours per week) is paid at double the regular wage. Taxes are removed based on the total earned, including overtime. Your job is to compute the total pay for that paycheck and return it in the : getPay method. Implement the Paycheck class using the template provided here: Paycheck.java I. Assume all input values are valid and non-negative. Examples: Paycheck pl new Paycheck (10.5, 42, 0); pl.getPay (); // returns 462.0 Paycheck p2 new Paycheck (10.5, 42, 10); p2.getPay (); // returns 415.8 Paycheck p3 new Paycheck (10.5, 40, 10); p3. getPay (); // returns 378 Paycheck p4 new Paycheck (10.5, 33.2, 25); p4.getPay (); // returns 261.45 Paycheck p5 new Paycheck (100.33, 65.5, 20); p5.getPay (); // returns 7304.024
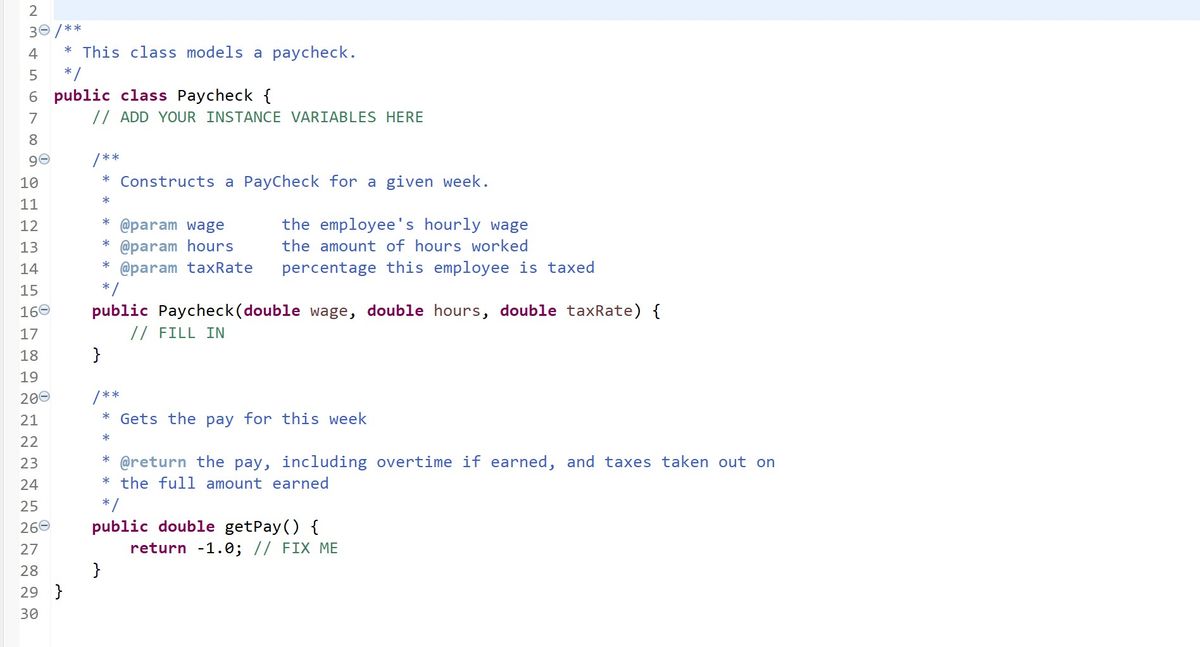
Extracted text: 2 30 /** 4 * This class models a paycheck. */ 6 public class Paycheck { 7 // ADD YOUR INSTANCE VARIABLES HERE 8. / ** 10 Constructs a PayCheck for a given week. * 11 the employee's hourly wage * @param wage * @param hours * @param taxRate */ 12 13 the amount of hours worked 14 percentage this employee is taxed 15 public Paycheck(double wage, double hours, double taxRate) { // FILL IN } 160 17 18 19 200 /** 21 * Gets the pay for this week * 22 * @return the pay, including overtime if earned, and taxes taken out on * the full amount earned 23 24 25 */ public double getPay () { return -1.0; // FIX ME } 260 27 28 29 } 30