#include "card.h"
#include "deck.h"
#include "hand.h"
using namespace std;
/************************************************************
* FunctionName *
* Function description *
* *
* *
************************************************************/
int main()
{
string repeat = "Y";
Deck myDeck;
Hand myHand;
string exchangeCards;
while (repeat == "Y" || repeat == "y")
{
cout <>
myHand.newHand(myDeck);
myHand.print();
cout <>
cout < "would="" you="" like="" to="" exchange="" any="" cards?="" [y="" n]:="">
getline(cin, exchangeCards);
while (exchangeCards != "Y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n")
{
cout < "please="" enter="" y="" or="" n="" only:="">
getline(cin, exchangeCards);
}
if(exchangeCards = "Y" || exchangeCards = "y")
{
myHand.exchangeCards(myDeck);
}
cout <>
myHand.print();
cout <>
myDeck.reset(); // Resets the deck for a new game
cout < "play="" again?="" [y="" n]:="">
getline(cin, repeat);
while (repeat != "Y" && repeat != "y" && repeat != "N" && repeat != "n")
{
cout < "please="" enter="" y="" or="" n="" only:="">
getline(cin, repeat);
}
}
return 0;
}
deck.h:
deck.h
#ifndef DECK_H
#define DECK_H
#include
#include // srand(), rand()
#include // time()
#include "card.h" // Include card header file here
using namespace std;
class Deck
{
public:
// class Constructor
Deck();
// Reset deck to new state (completely undealt)
void resetDeck();
// Print all cards in the undealt deck
void printUndealtDeck();
// Print all cards in the dealt deck
void printDealtDeck();
// Get size of the undealt deck
const int getSizeUndealtDeck();
// Get size of the dealt deck
const int getSizeDealtDeck();
// Deal a single card
Card dealCard(); // Is the dealCard() here an accessor or mutator function???
private:
vector m_undealtDeck; // Undealt cards
vector m_dealtDeck; // Dealt cards
};
#endif
// card.h
#ifndef CARD_H
#define CARD_H
#include
#include
using namespace std;
// Sources: https://en.wikipedia.org/wiki/Standard_52-card_deck
// https://en.wikipedia.org/wiki/Pip_(counting)
const string pips[] = {"Ace", "Two", "Three", "Four", "Five",
"Six", "Seven", "Eight", "Nine", "Ten",
"Jack", "Queen", "King"};
const string suits[] = {"Hearts", "Spades", "Clubs", "Diamonds"};
class Card
{
public:
// Get card value
int get();
// Set card value
void set(int value);
string getPip();
string getSuit();
// Print card value
void print();
private:
int m_cardValue;
};
#endif
hand.h:
hand.h
#ifndef HAND_H
#define HAND_H
#include
#include "card.h" // Include card header file here
#include "deck.h" // Include deck header file here
using namespace std;
const int NUM_CARDS_ON_HAND = 5;
class Hand
{
public:
// Put new card from deck into given location in a hand
void newCard(Deck& deck, int location);
// Get all new cards
void newHand(Deck& deck);
// Determine cards to exchange and exchange them in a hand
void exchangeCards(Deck& deck);
// Print the hand
void print();
private:
Card m_hand[NUM_CARDS_ON_HAND]; // A hand consists of 5 cards
};
#endif
How do I fix the error on line 62? I know C++ and know how to debug a program but this is a different book. I apollogize I am trying to understand it and this is the only way I'll how.
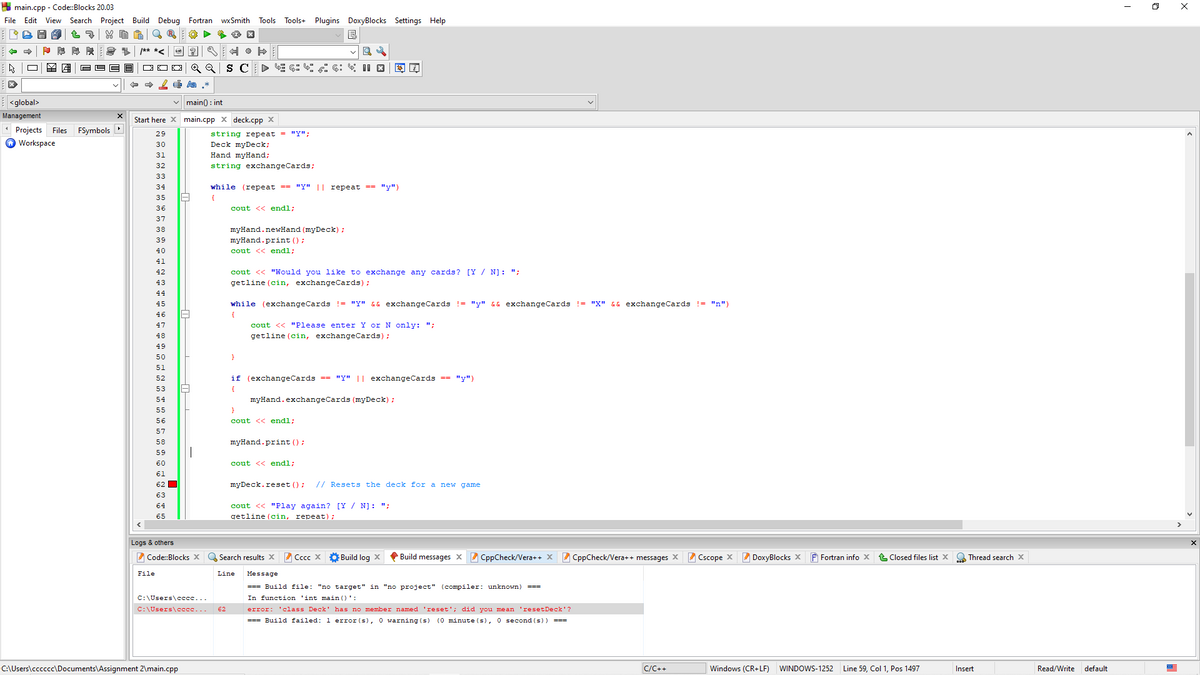
Extracted text: A main.cpp - Code:Blocks 20.03 X File Edit View Search Project Build Debug Fortran wxSmith Tools Tools+ Plugins DoxyBlocks Settings Help /** *< s="" c="" |=""> vmain) : int Management Start here X main.cpp x deck.cpp X Projects FSymbols Files string repeat = "Y"; Deck myDeck; 29 Workspace 30 31 Hand myHand; 32 string exchangeCards; 33 34 while (repeat == "Y" || repeat == "y") 35 36 cout < endl;="" 37="" 38="" myhand.newhand="" (mydeck)="" ;="" myhand.print="" ();="" cout="">< endl;="" 39="" 40="" 41="" 42="" cout="">< "would="" you="" like="" to="" exchange="" any="" cards?="" [y="" n]:="" ";="" 43="" getline="" (cin,="" exchangecards);="" 44="" 45="" while="" (exchangecards="" !="y" &&="" exchangecards="" !="y" &&="" exchangecards="" !="X" &&="" exchangecards="" !="n" )="" 46="" {="" 47="" cout="">< "please="" enter="" y="" or="" n="" only:="" ";="" 48="" getline="" (cin,="" exchangecards);="" 49="" 50="" 51="" 52="" if="" (exchangecards="=" "y"="" ||="" exchangecards="=" "y")="" 53="" {="" 54="" myhand.exchangecards="" (mydeck)="" ;="" 55="" 56="" cout="">< endl;="" 57="" 58="" myhand.print="" ();="" cout="">< endl;="" 61="" 62="" o="" mydeck.reset="" ()="" ;="" resets="" the="" deck="" for="" a="" new="" game="" 63="" cout="">< "play again? [y / n]: "; getline (cin, repeat); 64 65 logs & others 2 code:blocks x q search results x a cccc x ở build log x p build messages x cppcheck/vera++ x 2 cppcheck/vera++ messages x cscope x z doxyblocks x f fortran info x closed files list x q thread search x file line message === build file: "no target" in "no project" (compiler: unknown) === c:\users\cccc... in function 'int main ()': c:\users\ccee... 62 error: 'class deck' has no member named 'reset'; did you mean 'resetdeck'? === build failed: 1 error (s), 0 warning (s) (0 minute (s), 0 second (s)) === c:\users\cccccc\documents\assignment 2\main.cpp c/c++ windows (cr+lf) windows-1252 line 59, col 1, pos 1497 insert read/write default "play="" again?="" [y="" n]:="" ";="" getline="" (cin,="" repeat);="" 64="" 65="" logs="" &="" others="" 2="" code:blocks="" x="" q="" search="" results="" x="" a="" cccc="" x="" ở="" build="" log="" x="" p="" build="" messages="" x="" cppcheck/vera++="" x="" 2="" cppcheck/vera++="" messages="" x="" cscope="" x="" z="" doxyblocks="" x="" f="" fortran="" info="" x="" closed="" files="" list="" x="" q="" thread="" search="" x="" file="" line="" message="==" build="" file:="" "no="" target"="" in="" "no="" project"="" (compiler:="" unknown)="==" c:\users\cccc...="" in="" function="" 'int="" main="" ()':="" c:\users\ccee...="" 62="" error:="" 'class="" deck'="" has="" no="" member="" named="" 'reset';="" did="" you="" mean="" 'resetdeck'?="==" build="" failed:="" 1="" error="" (s),="" 0="" warning="" (s)="" (0="" minute="" (s),="" 0="" second="" (s))="==" c:\users\cccccc\documents\assignment="" 2\main.cpp="" c/c++="" windows="" (cr+lf)="" windows-1252="" line="" 59,="" col="" 1,="" pos="" 1497="" insert="" read/write=""> "play again? [y / n]: "; getline (cin, repeat); 64 65 logs & others 2 code:blocks x q search results x a cccc x ở build log x p build messages x cppcheck/vera++ x 2 cppcheck/vera++ messages x cscope x z doxyblocks x f fortran info x closed files list x q thread search x file line message === build file: "no target" in "no project" (compiler: unknown) === c:\users\cccc... in function 'int main ()': c:\users\ccee... 62 error: 'class deck' has no member named 'reset'; did you mean 'resetdeck'? === build failed: 1 error (s), 0 warning (s) (0 minute (s), 0 second (s)) === c:\users\cccccc\documents\assignment 2\main.cpp c/c++ windows (cr+lf) windows-1252 line 59, col 1, pos 1497 insert read/write default>