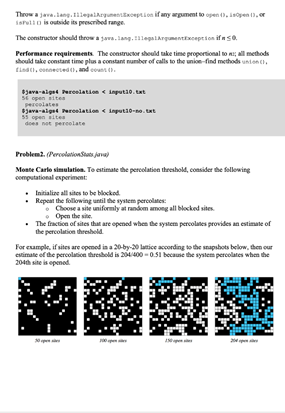
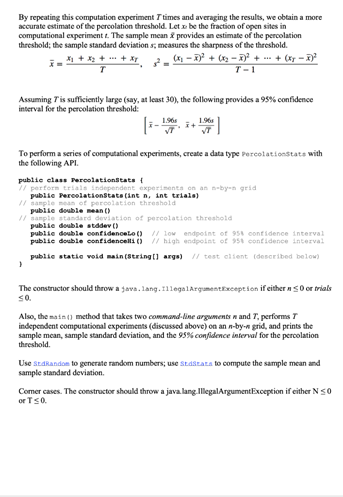
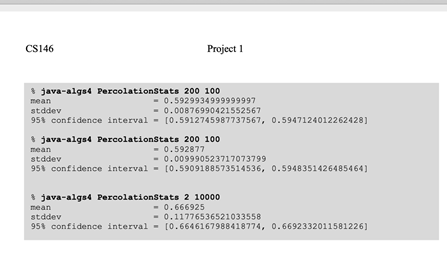
For Percolation.Java
import edu.princeton.cs.algs4.In;
import edu.princeton.cs.algs4.StdOut;
import edu.princeton.cs.algs4.WeightedQuickUnionUF;
// Models an N-by-N percolation system.
public class Percolation {
// Create an N-by-N grid, with all sites blocked.
//...
public Percolation(int N) {
//...
}
// Open site (row, col) if it is not open already.
public void open(int row, int col) {
//...
}
// Is site (row, col) open?
public boolean isOpen(int row, int col) {
//...
return false;
}
// Is site (row, col) full?
public boolean isFull(int row, int col) {
//...
return false;
}
// Number of open sites.
public int numberOfOpenSites() {
//...
return 0;
}
// Does the system percolate?
public boolean percolates() {
//...
return false;
}
// An integer ID (1...N) for site (row, col).
private int encode(int row, int col) {
//...
return 0;
}
// Test client. [DO NOT EDIT]
public static void main(String[] args) {
String filename = args[0];
In in = new In(filename);
int N = in.readInt();
Percolation perc = new Percolation(N);
while (!in.isEmpty()) {
int i = in.readInt();
int j = in.readInt();
perc.open(i, j);
}
StdOut.println(perc.numberOfOpenSites() + " open sites");
if (perc.percolates()) {
StdOut.println("percolates");
}
else {
StdOut.println("does not percolate");
}
// Check if site (i, j) optionally specified on the command line
// is full.
if (args.length == 3) {
int i = Integer.parseInt(args[1]);
int j = Integer.parseInt(args[2]);
StdOut.println(perc.isFull(i, j));
}
}
}
For Percolationstats.java
import edu.princeton.cs.algs4.StdOut;
import edu.princeton.cs.algs4.StdRandom;
import edu.princeton.cs.algs4.StdStats;
// Estimates percolation threshold for an N-by-N percolation system.
public class PercolationStats {
...
// Perform T independent experiments (Monte Carlo simulations) on an
// N-by-N grid.
public PercolationStats(int N, int T) {
...
}
// Sample mean of percolation threshold.
public double mean() {
...
}
// Sample standard deviation of percolation threshold.
public double stddev() {
...
}
// Low endpoint of the 95% confidence interval.
public double confidenceLow() {
...
}
// High endpoint of the 95% confidence interval.
public double confidenceHigh() {
...
}
// Test client. [DO NOT EDIT]
public static void main(String[] args) {
int N = Integer.parseInt(args[0]);
int T = Integer.parseInt(args[1]);
PercolationStats stats = new PercolationStats(N, T);
StdOut.printf("mean = %f\n", stats.mean());
StdOut.printf("stddev = %f\n", stats.stddev());
StdOut.printf("confidenceLow = %f\n", stats.confidenceLow());
StdOut.printf("confidenceHigh = %f\n", stats.confidenceHigh());
}
}
For PercolationVisualizer.java
/* *****************************************************************************
* Compilation: javac-algs4 PercolationVisualizer.java
* Execution: java-algs4 PercolationVisualizer input.txt
* Dependencies: Percolation.java
*
* This program takes the name of a file as a command-line argument.
* From that file, it
*
* - Reads the grid size n of the percolation system.
* - Creates an n-by-n grid of sites (intially all blocked)
* - Reads in a sequence of sites (row, col) to open.
*
* After each site is opened, it draws full sites in light blue,
* open sites (that aren't full) in white, and blocked sites in black,
* with with site (0, 0) in the upper left-hand corner.
*
**************************************************************************** */
import edu.princeton.cs.algs4.In;
import edu.princeton.cs.algs4.StdDraw;
import java.awt.Font;
public class PercolationVisualizer {
// delay in miliseconds (controls animation speed)
private static final int DELAY = 100;
// draw n-by-n percolation system
public static void draw(Percolation percolation, int n) {
StdDraw.clear();
StdDraw.setPenColor(StdDraw.BLACK);
StdDraw.setXscale(-0.05 * n, 1.05 * n);
StdDraw.setYscale(-0.05 * n, 1.05 * n); // leave a 5% border
StdDraw.filledSquare(n / 2.0, n / 2.0, n / 2.0);
// draw n-by-n grid
for (int row = 0; row <>
for (int col = 0; col <>
if (percolation.isFull(row, col)) {
StdDraw.setPenColor(StdDraw.BOOK_LIGHT_BLUE);
}
else if (percolation.isOpen(row, col)) {
StdDraw.setPenColor(StdDraw.WHITE);
}
else {
StdDraw.setPenColor(StdDraw.BLACK);
}
StdDraw.filledSquare(col + 0.5, n - row - 0.5, 0.45);
}
}
// write status text
StdDraw.setFont(new Font("SansSerif", Font.PLAIN, 12));
StdDraw.setPenColor(StdDraw.BLACK);
StdDraw.text(0.25 * n, -0.025 * n, percolation.numberOfOpenSites() + " open sites");
if (percolation.percolates()) StdDraw.text(0.75 * n, -0.025 * n, "percolates");
else StdDraw.text(0.75 * n, -0.025 * n, "does not percolate");
}
private static void simulateFromFile(String filename) {
In in = new In(filename);
int n = in.readInt();
Percolation percolation = new Percolation(n);
// turn on animation mode
StdDraw.enableDoubleBuffering();
// repeatedly read in sites to open and draw resulting system
draw(percolation, n);
StdDraw.show();
StdDraw.pause(DELAY);
while (!in.isEmpty()) {
int row = in.readInt();
int col = in.readInt();
percolation.open(row, col);
draw(percolation, n);
StdDraw.show();
StdDraw.pause(DELAY);
}
}
public static void main(String[] args) {
String filename = args[0];
simulateFromFile(filename);
}
}
}