error on python code: File "/root/sandbox/MyMotorcycleClassProgram.py", line 11, in
motorcycleOne = Motorcycle(90.0)
TypeError: Motorcycle.__init__() takes 1 positional argument but 2 were given
from Vehicle import Vehicle
# Write the Motorcycle class here
class Motorcycle(Vehicle):
def__init__(self):
Vehicle.__init__(self)
self._sidecar = sidecar
defset_sidecar(self,sC):
self._sidecar = sidecar
defget_sidecar(self):
returnself._sidecar
defaccelerate(self,mph):
ifself._speed + mph >self._max_speed:
self._speed =self._speed + mph
else:
print("This motorcycle cannot go that fast.")
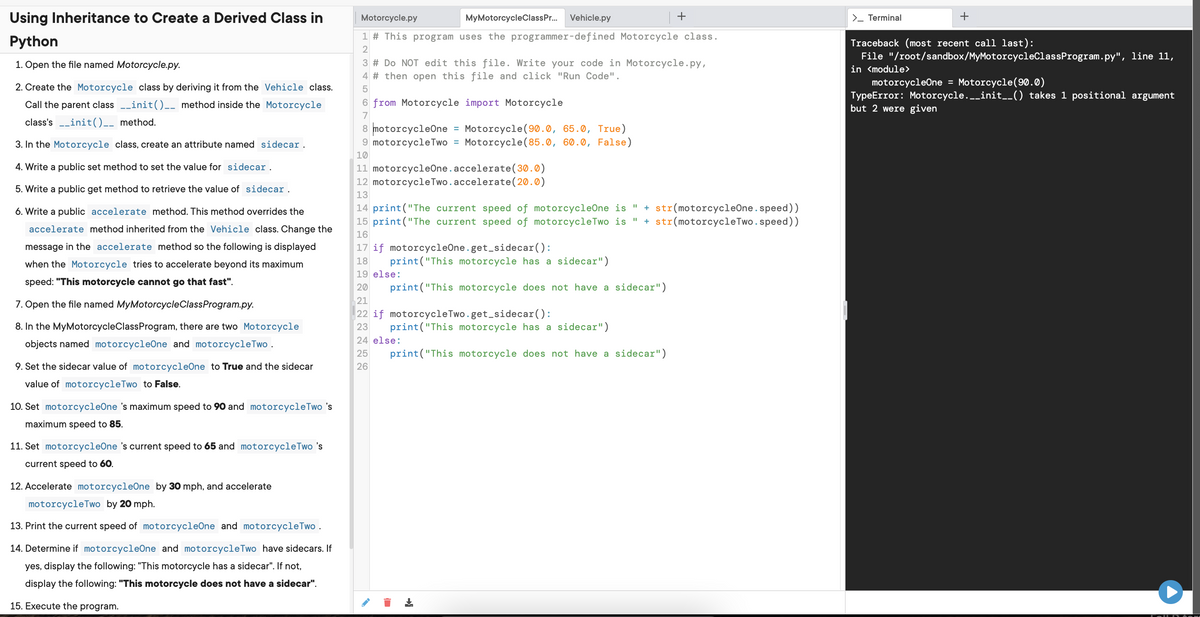
2 3 # Do NOT edit this file. Write your code in Motorcycle.py, 4 # then open this file and click "Run Code". 1. Open the file named Motorcycle.py. Motorcycle(90.0) motorcycleOne TypeError: Motorcycle._init_-() takes 1 positional argument but 2 were given 2. Create the Motorcycle class by deriving it from the Vehicle class. Call the parent class --init()-- method inside the Motorcycle 6 from Motorcycle import Motorcycle 7 class's -_init()-- method. 8 motorcycleOne 9 motorcycleTwo = Motorcycle(85.0, 60.0, False) = Motorcycle(90.0, 65.0, True) 3. In the Motorcycle class, create an attribute named sidecar. 10 4. Write a public set method to set the value for sidecar. 11 motorcycleOne.accelerate(30.0) 12 motorcycle Two.accelerate(20.0) 5. Write a public get method to retrieve the value of sidecar. 13 14 print("The current speed of motorcycleOne is " 15 print ("The current speed of motorcycleTwo is " + str(motorcycleOne.speed)) + str(motorcycleTwo.speed)) 6. Write a public accelerate method. This method overrides the accelerate method inherited from the Vehicle class. Change the 16 message in the accelerate method so the following is displayed 17 if motorcycleOne.get_sidecar(): when the Motorcycle tries to accelerate beyond its maximum 18 print("This motorcycle has a sidecar") 19 else: speed: "This motorcycle cannot go that fast". 20 print("This motorcycle does not have a sidecar") 21 7. Open the file named MyMotorcycleClassProgram.py. 22 if motorcycleTwo.get_sidecar(): 8. In the MyMotorcycleClassProgram, there are two Motorcycle 23 print("This motorcycle has a sidecar") 24 else: objects named motorcycleOne and motorcycleTwo . 25 print("This motorcycle does not have a sidecar") 9. Set the sidecar value of motorcycleOne to True and the sidecar 26 value of motorcycleTwo to False. 10. Set motorcycleOne 's maximum speed to 90 and motorcycleTwo 's maximum speed to 85. 11. Set motorcycleOne 's current speed to 65 and motorcycleTwo 's current speed to 60. 12. Accelerate motorcycleOne by 30 mph, and accelerate motorcycleTwo by 20 mph. 13. Print the current speed of motorcycleOne and motorcycleTwo . 14. Determine if motorcycleOne and motorcycleTwo have sidecars. If yes, display the following: "This motorcycle has a sidecar". If not, display the following: "This motorcycle does not have a sidecar". 15. Execute the program. "/>
Extracted text: Using Inheritance to Create a Derived Class in Motorcycle.py MyMotorcycleClassPr. Vehicle.py >_ Terminal 1 # This program uses the programmer-defined Motorcycle class. Python Traceback (most recent call last): File "/root/sandbox/MyMotorcycleClassProgram.py", line 11, in 2 3 # Do NOT edit this file. Write your code in Motorcycle.py, 4 # then open this file and click "Run Code". 1. Open the file named Motorcycle.py. Motorcycle(90.0) motorcycleOne TypeError: Motorcycle._init_-() takes 1 positional argument but 2 were given 2. Create the Motorcycle class by deriving it from the Vehicle class. Call the parent class --init()-- method inside the Motorcycle 6 from Motorcycle import Motorcycle 7 class's -_init()-- method. 8 motorcycleOne 9 motorcycleTwo = Motorcycle(85.0, 60.0, False) = Motorcycle(90.0, 65.0, True) 3. In the Motorcycle class, create an attribute named sidecar. 10 4. Write a public set method to set the value for sidecar. 11 motorcycleOne.accelerate(30.0) 12 motorcycle Two.accelerate(20.0) 5. Write a public get method to retrieve the value of sidecar. 13 14 print("The current speed of motorcycleOne is " 15 print ("The current speed of motorcycleTwo is " + str(motorcycleOne.speed)) + str(motorcycleTwo.speed)) 6. Write a public accelerate method. This method overrides the accelerate method inherited from the Vehicle class. Change the 16 message in the accelerate method so the following is displayed 17 if motorcycleOne.get_sidecar(): when the Motorcycle tries to accelerate beyond its maximum 18 print("This motorcycle has a sidecar") 19 else: speed: "This motorcycle cannot go that fast". 20 print("This motorcycle does not have a sidecar") 21 7. Open the file named MyMotorcycleClassProgram.py. 22 if motorcycleTwo.get_sidecar(): 8. In the MyMotorcycleClassProgram, there are two Motorcycle 23 print("This motorcycle has a sidecar") 24 else: objects named motorcycleOne and motorcycleTwo . 25 print("This motorcycle does not have a sidecar") 9. Set the sidecar value of motorcycleOne to True and the sidecar 26 value of motorcycleTwo to False. 10. Set motorcycleOne 's maximum speed to 90 and motorcycleTwo 's maximum speed to 85. 11. Set motorcycleOne 's current speed to 65 and motorcycleTwo 's current speed to 60. 12. Accelerate motorcycleOne by 30 mph, and accelerate motorcycleTwo by 20 mph. 13. Print the current speed of motorcycleOne and motorcycleTwo . 14. Determine if motorcycleOne and motorcycleTwo have sidecars. If yes, display the following: "This motorcycle has a sidecar". If not, display the following: "This motorcycle does not have a sidecar". 15. Execute the program.
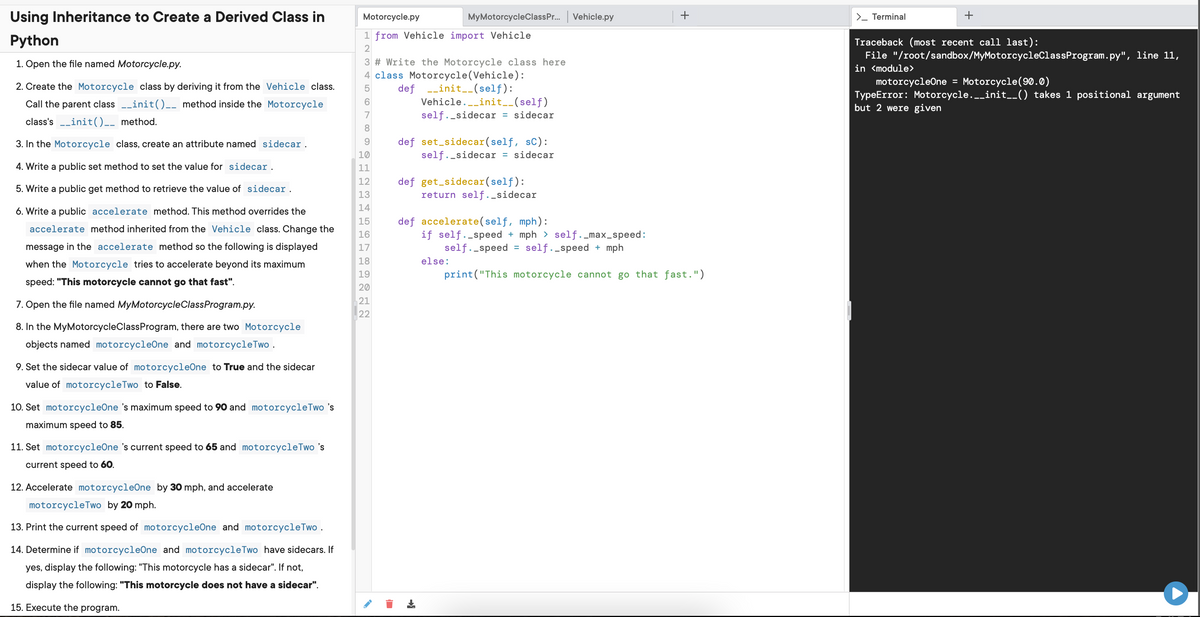
2 3 # Write the Motorcycle class here 4 class Motorcycle(Vehicle): def --init_-(self): Vehicle._init__(self) 1. Open the file named Motorcycle.py. motorcycleOne = Motorcycle(90.0) TypeError: Motorcycle._init_-() takes 1 positional argument but 2 were given 2. Create the Motorcycle class by deriving it from the Vehicle class. Call the parent class --init()-- method inside the Motorcycle 7 self._sidecar = sidecar class's _init()_- method. 8. def set_sidecar(self, sC): self._sidecar = sidecar 3. In the Motorcycle class, create an attribute named sidecar. 9. 10 4. Write a public set method to set the value for sidecar . 11 def get_sidecar(self): return self._sidecar 12 5. Write a public get method to retrieve the value of sidecar. 13 14 6. Write a public accelerate method. This method overrides the def accelerate(self, mph): if self._speed + mph > self._max_speed: self._speed = self._speed + mph 15 accelerate method inherited from the Vehicle class. Change the 16 message in the accelerate method so the following is displayed 17 18 else: when the Motorcycle tries to accelerate beyond its maximum 19 print("This motorcycle cannot go that fast.") speed: "This motorcycle cannot go that fast". 20 7. Open the file named MyMotorcycleClassProgram.py. 21 22 8. In the MyMotorcycleClassProgram, there are two Motorcycle objects named motorcycleOne and motorcycleTwo . 9. Set the sidecar value of motorcycleOne to True and the sidecar value of motorcycleTwo to False. 10. Set motorcycleOne 's maximum speed to 90 and motorcycleTwo 's maximum speed to 85. 11. Set motorcycleOne 's current speed to 65 and motorcycleTwo 's current speed to 60. 12. Accelerate motorcycleOne by 30 mph, and accelerate motorcycleTwo by 20 mph. 13. Print the current speed of motorcycleOne and motorcycleTwo . 14. Determine if motorcycleOne and motorcycleTwo have sidecars. If yes, display the following: "This motorcycle has a sidecar". If not, display the following: "This motorcycle does not have a sidecar". 15. Execute the program. "/>
Extracted text: Using Inheritance to Create a Derived Class in Motorcycle.py Vehicle.py + + MyMotorcycleClassPr. >_ Terminal 1 from Vehicle import Vehicle Python Traceback (most recent call last): File "/root/sandbox/MyMotorcycleClassProgram.py", line 11, in 2 3 # Write the Motorcycle class here 4 class Motorcycle(Vehicle): def --init_-(self): Vehicle._init__(self) 1. Open the file named Motorcycle.py. motorcycleOne = Motorcycle(90.0) TypeError: Motorcycle._init_-() takes 1 positional argument but 2 were given 2. Create the Motorcycle class by deriving it from the Vehicle class. Call the parent class --init()-- method inside the Motorcycle 7 self._sidecar = sidecar class's _init()_- method. 8. def set_sidecar(self, sC): self._sidecar = sidecar 3. In the Motorcycle class, create an attribute named sidecar. 9. 10 4. Write a public set method to set the value for sidecar . 11 def get_sidecar(self): return self._sidecar 12 5. Write a public get method to retrieve the value of sidecar. 13 14 6. Write a public accelerate method. This method overrides the def accelerate(self, mph): if self._speed + mph > self._max_speed: self._speed = self._speed + mph 15 accelerate method inherited from the Vehicle class. Change the 16 message in the accelerate method so the following is displayed 17 18 else: when the Motorcycle tries to accelerate beyond its maximum 19 print("This motorcycle cannot go that fast.") speed: "This motorcycle cannot go that fast". 20 7. Open the file named MyMotorcycleClassProgram.py. 21 22 8. In the MyMotorcycleClassProgram, there are two Motorcycle objects named motorcycleOne and motorcycleTwo . 9. Set the sidecar value of motorcycleOne to True and the sidecar value of motorcycleTwo to False. 10. Set motorcycleOne 's maximum speed to 90 and motorcycleTwo 's maximum speed to 85. 11. Set motorcycleOne 's current speed to 65 and motorcycleTwo 's current speed to 60. 12. Accelerate motorcycleOne by 30 mph, and accelerate motorcycleTwo by 20 mph. 13. Print the current speed of motorcycleOne and motorcycleTwo . 14. Determine if motorcycleOne and motorcycleTwo have sidecars. If yes, display the following: "This motorcycle has a sidecar". If not, display the following: "This motorcycle does not have a sidecar". 15. Execute the program.