Develop aclass libraryfor a series of evaluations given to students in different courses. Note that this is not a running project: it's aclass library.
There are three different kinds of evaluations being modeled in this class library:
- Graded Evaluations
- Modeled by the class GradedEval.
- A graded evaluation is an evaluation where the student submits work and the work is graded by the professor or instructor.
- A graded evaluation has fields for title, weight (how much of the final grade an evaluation is worth), maximum grade (how much an evaluation is out of), and actual grade (the actual grade the student received).
- Example: a student writes a mid term exam out of 67 that is worth 25% of their final grade and they get 60/67 on that exam. The GradedEval object's title would be "Mid Term Exam", the weight would be 25, the maximum grade would be 67 and the actual grade would be 60. In this example, the percentage achieved towards the student's final grade is 60/67 * 25 = 22.4
GradedEval Example |
---|
title |
Mid Term Exam |
weight |
25 |
grade |
60 |
maxGrade |
67 |
calculated achieved weight |
60 / 67 * 25 = 22.4 |
- Check-Off Evaluation
- Modeled by the class CheckedEval.
- A check-off evaluation is an evauation where the student does an exercise or other work and the work is checked off as "complete" by the professor or instructor.
- A checked-off evaluation has fields for title, weight (how much of the final grade an evaluation is worth), maximum grade (how much an evaluation is out of), actual grade (the actual grade the student received), and completion (a boolean that determines whether or not the evaluation was "checked off" as complete).
- Example: a student does the first in-class exercise that is out of 5 and worth 2% of the final grade. The student completes the in-class exercise. The CheckedEval object's title would be set to "In-Class 1", the weight would be 2, the maximum grade would be 5 and the actual grade would be set to 5 as soon as the complete field is set to true (and would be set to 0 if the complete field was set back to false). In this example, when the assignment is complete, the percentage achieved towards the student's final grade is 5/5 * 2 = 2%
CheckedEval Example |
---|
title |
In-Class 1 |
weight |
2 |
grade |
5 |
maxGrade |
5 |
complete |
true |
calculated achieved weight |
5 / 5 * 2 = 2 |
- Field Study Evaluation
- Modeled by the class FieldEval.
- A field study evaluation occurs when a student goes out into the field for a period of time and performs industry work related to the field of study.
- A field study evaluation has fields for the title, weight, performance score (out of 100%, determined by the professor or instructor based on on-site performance evaluation), and employer evaluation score (out of 100%, a score submitted by the employer that scores the student's performance during the field study).
- Example: a student takes an ECE course where they do a field study worth 50% of their course grade at a selected child care centre called "Happy Jellybean Day Care". The professor performs a field visit to evaluate the student's performance and gives them a score of 75%. After the field study is complete, the employer at the child care centre gives the student a performance score of 90%. The FieldEval object's title would be set to "Happy Jellybean Day Care", the weight to 50, the performance to 75, and the employer performance to 90. Since the two scores are percentages, it is assumed that they're out of 100. In this example, the percentage achieved towards the student's final grade is (75 + 90) / 200 * 50 = 41.3%
FieldEval Example |
---|
title |
Happy Jellybean Day Care |
weight |
50 |
performance |
75 |
employerEval |
90 |
calculated achieved weight |
(75 + 90) / 200 * 50 = 41.3 |
Project Specs
Project Name:A2_LoginName(where LoginName is your own sheridan login name).
Package Name:loginname(your own login name, in lower-case letters). Do not use prog24178.loginname or anything else, just loginname.
Note once again that this is aclass library. It is not a running project.
Of course you might wish to test your code. You can do this by creating a second project where you add your Evaluations library. However, do not submit this application project.
Failure to follow the above instructions could result in penalites.I'll also be testing your assignment by adding your library's .jar file to a new application and using JUnit (which relies on having your package and class names following the specifications above).
Your classes must be related as depicted in the diagram below:
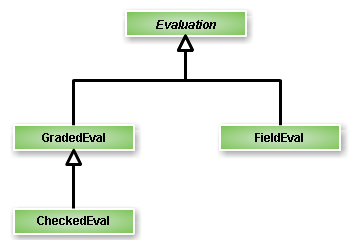
Assignment 2 Class Relationships
Notes:
Each class in your class library must have proper and complete JAVADOC documentation. You don't have to generate the JavaDocs, just have the comments in your code.
- Evaluation is an abstract class.
- It is up to you to determine what data members should go in each of the classes.
- All data members defined in a class require accessor and mutator methods. Mutator methods must throw the appropriate exception object with concise and informative error messages. Each data member's details are as follows (it is up to you to decide what member goes in which class):
- NOTE:the names and data types used below are the actual names and data types you must use. Failure to follow these conventions will result in your classes not working with the main application.
- title: String - the title of the evaluation. This can't be empty and it can't be a null object. The default value is "TBD" (TBD = To Be Determined).
- weight: double - the percentage of the final grade this evaluation is worth (for example, if an evaluation is worth 10% of the final grade, then weight would be 10.0). The weight can't be 0 or less and the default is 10.
- grade: double - the grade the student received on the evaluation. The grade can't be less than 0. The default value is 0.
- maxGrade: double - the number of marks an evaluation is out of (for example, if a student gets 20/25 on an assignment, 25 is the maximum grade). The maximum grade must be greater than 0, and the default value is 10.
- complete: boolean - true if the evaluation is marked as complete and false if the evaluation is marked as incomplete. Validation for boolean members is not required. The default value is false. When an evaluation is marked as complete, the actual grade is set to the maximum grade for this evaluation. For example, if an assignment is out of 15, the actual grade is set to 15 when the assignment is marked as complete. It's rare, but it's possible for an assignment to be later changed to incomplete, in which case the actual grade would be set back to 0.
- performance: double - the performance score the professor or instructor assigns to the student for their performance on a field study evaluation. This score must be 0 or greater. There is no maximum, although for testing purposes it might be helpful to note that it's usually out of 100.
- employerEval: double - the performance score the employer assigns to the student for their performance on a field study evaluation. This score must be 0 or greater. There is no maximum, although for testing purposes it might be helpful to note that it's usually out of 100.
- Evaluation contains an abstract method called achievedWeight(). This method needs to be overridden in each of the other classes to calculate and return the percentage of the final grade the student has achieved for an evaluation as follows:
- GradedEval and CheckedEval: achievedWeight() returns the actual grade / maximum grade * the evaluation weight
- FieldEval: achievedWeight() returns the performance score and employer evaluation score added together, then divided by 200 and multiplied by the evaluation weight.
- Each class should have a String representation from implicit calls to toString() as follows:
- GradedEval:GradedEval - gg.g/mm.m(where gg.g is the actual grade and mm.m is the maximum grade, both of which are formatted to one decimal place)
- CheckedEval:CheckededEval - gg.g/mm.m (COMPLETE)(where gg.g is the actual grade and mm.m is the maximum grade, both of which are formatted to one decimal place; the value COMPLETE or INCOMPLETE in all upper-case is displayed in parenthesis after the maximum grade)
- FieldEval:FieldEval - pp.p, ee.e(where pp.p is the performance score and ee.e is the employer evaluation score, both formatted to one decimal place)
- Each class* should have three constructors:
- a default constructor
- a 2-param constructor that constructs a new object with a specific title and weight
- a multi-param constructor that takes values for each data member required to fully initialize an object. For example, GradedEval(title, weight, grade, maxGrade), CheckedEval(title, weight, grade, maxGrade, complete), and FieldEval(title, weight, performance, employeeEval). *Evaluation class doesn't need the multi-param constructor.