();
Path file = Paths.get("info2.txt");
Scanner sc = null;
try {
sc = new Scanner(new File(file.getFileName().toString()));
System.out.println("Information Read form file:");
while (sc.hasNext()) {
bigRecord = sc.nextLine();
System.out.println("Reading Record " + index + ": " + bigRecord);
bigRecordArray[index++] = modifyVowels(bigRecord, modifyName);
}
sc.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
}
System.out.println("Modified Records:");
printRecords(bigRecordArray, index - 1);
bubbleSort(bigRecordArray, index - 1);
createLinkedList(list, bigRecordArray, index);
printList(list);
aNumber = rand.nextInt(index);
System.out.println("\nWill be removing record number: " + aNumber);
list.remove(aNumber);
printList(list);
aNumber = rand.nextInt(index);
System.out.println("\nWill be removing record number: " + aNumber);
list.remove(aNumber);
printList(list);
}
public static String modifyVowels(String fullName, String modifyName) {
if (fullName.length() == 0)
return modifyName;
else {
if (fullName.charAt(0) == '0')
modifyName = modifyName + "a";
else if (fullName.charAt(0) == '1')
modifyName = modifyName + "e";
else if (fullName.charAt(0) == '2')
modifyName = modifyName + "i";
else if (fullName.charAt(0) == '3')
modifyName = modifyName + "o";
else if (fullName.charAt(0) == '4')
modifyName = modifyName + "u";
else if (fullName.charAt(0) == '5')
modifyName = modifyName + "y";
else
modifyName = modifyName + fullName.charAt(0);
return modifyVowels(fullName.substring(1), modifyName);
}
}
public static void printRecords(String[] fullName, int index) {
for (int i = 0; i <= index;="" i++)="">=>
System.out.println("Record " + i + ": " + fullName[i]);
}
}
static void bubbleSort(String[] arr, int n) {
String temp = "";
for (int j = 0; j < n;="">
{
for (int i = j + 1; i < n="" +="" 1;="">
{
if (arr[j].compareTo(arr[i]) > 0)
{
temp = arr[j];
arr[j] = arr[i];
arr[i] = temp;
}
}
}
System.out.println("After Sorting:");
printRecords(arr, n);
}
static void createLinkedList(List list, String[] fullName, int index) {
for (int i = 0; i < index;="" i++)="">
list.add(fullName[i]);
}
}
static void printList(List list) {
System.out.println("\nLinked list records:");
int i = 0;
ListIterator iterator = list.listIterator();
while (iterator.hasNext())
System.out.println("Record " + i++ + ": " + iterator.next());
}
}
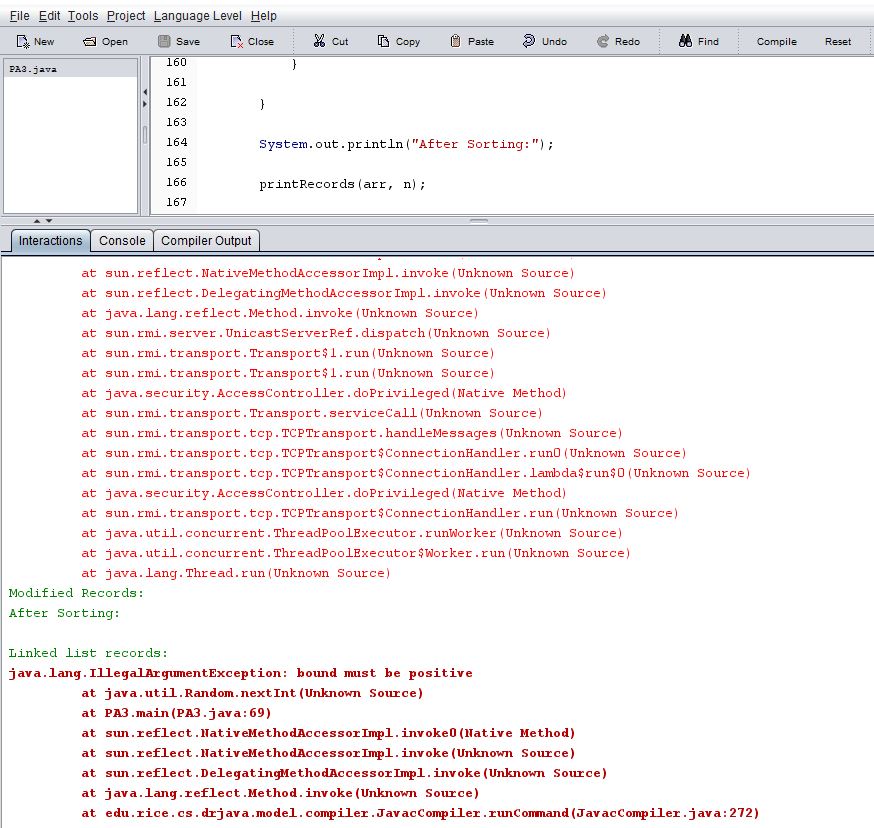
Extracted text: File Edit Tools Project Language Level Help LA New a Open L Close % Cut O Copy A Find Save Paste Undo Redo Compile Reset 160 } PA3.java 161 162 163 System.out.println ("After Sorting: "); 164 165 printRecords (arr, n); 166 167 Interactions Console Compiler Output at sun.reflect. NativeMethodAccessor Impl.invoke (Unknown Source) at sun.reflect. DelegatingMethodAccessor Impl.invoke (Unknown Source) at java.lang.reflect.Method. invoke (Unknown Source) at sun.rmi.server. UnicastServerRef.dispatch (Unknown Source) at sun.rmi.transport. Transport$1.run (Unknown Source) at sun.rmi. transport. Transport$1.run (Unknown Source) at java.security. AccessController.doPrivileged (Native Method) at sun.rmi.transport. Transport.serviceCall(Unknown Source) at sun.rmi.transport.tcp. TCPTransport.handleMessages (Unknown Source) at sun.rmi. transport.tcp. TCPTransport$ConnectionHandler.run0 (Unknown Source) at sun.rmi.transport.tcp. TCPTransport$ConnectionHandler.lambdaşrun$0 (Unknown Source) at java.security.AccessController.doPrivileged (Native Method) at sun.rmi.transport.tcp.TCPTransport$ConnectionHandler.run (Unknown Source) at java.util.concurrent. ThreadPoolExecutor.runWorker (Unknown Source) at java.util.concurrent. ThreadPoolExecutor $Worker.run (Unknown Source) at java.lang. Thread.run (Unknown Source) Modified Records: After Sorting: Linked list records: java.lang. IllegalArgumentException: bound must be positive at java.util. Random.next Int (Unknown Source) at PA3.main (PA3.java:69) at sun.reflect.NativeMethodAccessor Impl. invoke0 (Native Method) at sun.reflect.NativeMethodAccessor Impl.invoke (Unknown Source) at sun.reflect.DelegatingMethodAccessor Impl.invoke (Unknown Source) at java.lang.reflect.Method. invoke (Unknown Source) at edu.rice.cs.drjava.model.compiler.JavacCompiler.runcommand (JavacCompiler.java:272)