void printArray(int *arr, int size){
int i;
if (arr != NULL)
{
for( i = 0; i < size;="" i++)="">
// Print each element out
printf("%d", *(arr+i));
//Print addresses of each element
printf("%p", (arr+i));
//Printing a new line
printf("\n");
}
}
}
int main() {
// Allows user to specify the original array size, stored in variable n1.
printf("Enter original array size: ");
int n1 = 0;
scanf("%d", &n1);
//a- Create a new array *a1 of n1 ints using malloc
int *a1 = malloc(n1 * sizeof(int));
//b- Remove comments for if statment below
//b- Set each element in a1 to 100 + indexValue ??
if(a1 == NULL)
{
printf("Error! memory not allocated.");
exit(0);
}
int i;
for (i = 0; i < n1;="">
{
a1[i] = 100 + i;
}
printf("Printing the first array allocated using malloc\n");
//c- Print each element and addresses out (to make sure things look right)
printArray(a1, n1);
// User specifies the new array size, stored in variable n2.
printf("\nEnter new array size: ");
int n2 = 0;
scanf("%d", &n2);
//d- Dynamically change the array a1 to size n2
a1 = malloc (n2 * sizeof(int));
//e- Remove comments for if statment below
if(a1 == NULL)
{
printf("Error! memory not allocated.");
exit(0);
}
//e- If the new array is a larger size, set all new members to 200 + indexValue.
if (n2 > n1)
{
int i;
for (i = 0; i < n2;="">
{
a1[i] = 100 + i;
}
}
printf("Printing the reallocated array: \n");
//f- Print each element and addresses out (to make sure things look right)
printArray(a1, n2);
//g-Free the allocated memory for a1
free(a1);
a1 = NULL;
printf("\nEnter new array size to be initialized with 0: ");
int n3 = 0;
scanf("%d", &n3);
//h- Remove comments for if statement
//h- Using calloc create a new array and assign it to a2
//with new array size, stored in variable n3.
int *a2 =calloc(n3, sizeof(int));
if(a2 == NULL)
{
printf("Error! memory not allocated.");
exit(0);
}
printf("Printing the array created using calloc: \n");
//i- Print array a2 with size n3
printArray(a2, n3);
//j- Print array a1 again, How you can fix this problem
printf("Printing deallocated array a1: \n");
printArray(a1, n2);
// Done with arrays now, free the and assign NULL
free(a2);
a1 = NULL;
a2 = NULL;
printf("Program Successfully Completed!");
return 0;
}
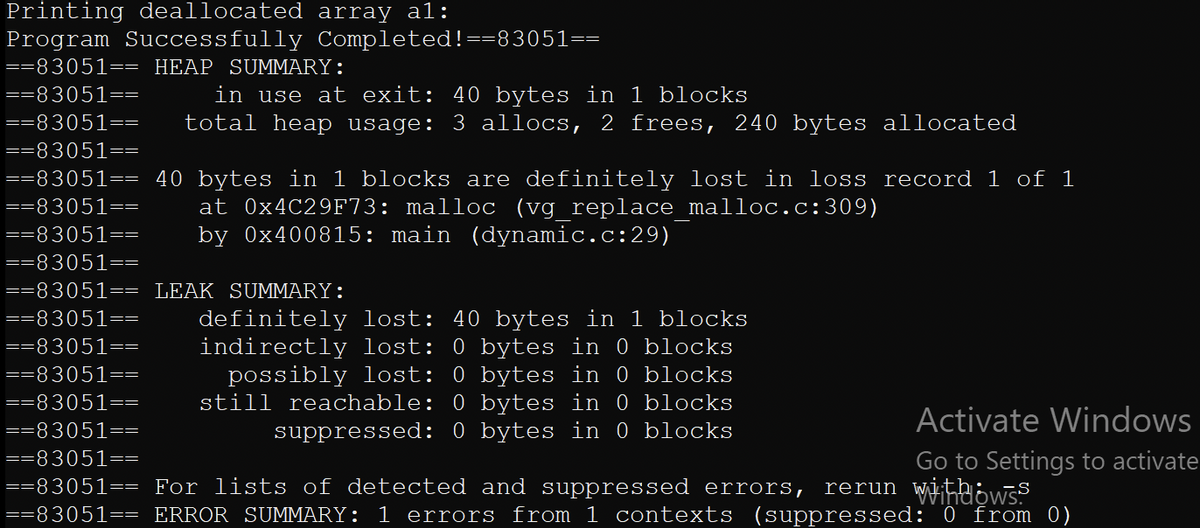
Extracted text: Printing deallocated array al: Program Successfully Completed!==83051== ==83051== HEAP SUMMARY: in use at exit: 40 bytes in 1 blocks total heap usage: 3 allocs, 2 frees, 240 bytes allocated ==83051== ==83051== ==83051== ==83051== 40 bytes in 1 blocks are definitely lost in loss record 1 of 1 ==83051== at 0×4C29F73: malloc (vg replace malloc.c:309) by 0x400815: main (dynamic.c:29) ==83051== ==83051== ==83051== LEAK SUMMARY: ==83051== definitely lost: 40 bytes in 1 blocks indirectly lost: 0 bytes in 0 blocks possibly lost: 0 bytes in 0 blocks still reachable: 0 bytes in 0 blocks suppressed: 0 bytes in 0 blocks ==83051== ==83051== ==83051== Activate Windows ==83051== ==83051== Go to Settings to activate ==83051== For lists of detected and suppressed errors, ==83051== ERROR SUMMARY: 1 errors from 1 contexts (suppressed: 0 from 0) rerun Wiheows.