1.1 Create aStudent Object project.
Create aStudent class and use the provided UML diagram to code the instance fields, constructors and methods.
Note:
The module mark is the average of the participation mark and the examination mark - calculate this using thecalcModuleMark() method.
ThedetermineStNumber() method should compile a student number with the first 3 letters of the student's name, a '#' symbol, and a 3-digit randomly generated number (make use of a for-loop to concatenate these 3-digits).
ThestNumber data field should be set in the constructor by calling thedetermineStNumber() method.
ThetoString() method must compile a string to display the student number, participation mark, examination mark, and module mark, formatted in table format.
Student number PMark EMark MMark
Mar#9186 81 64 72.00
1.2 Create atestStudents class. Do the following:
-Write a static method calleddisplay() which receives the Student object as a parameter, and displays the necessary heading, as well as thetoString() method from the Object class.
-In themain method, you should ask for the following input: the student's name, participation mark and an examination mark.
-Make use of a while-loop to input multiple student details, and use a sentinel control to stop input.
-After each student's details have been entered, instantiate an object of the Student class by calling the classes constructor.
-Use thedisplay() method to display the student's results before entering another student's details.
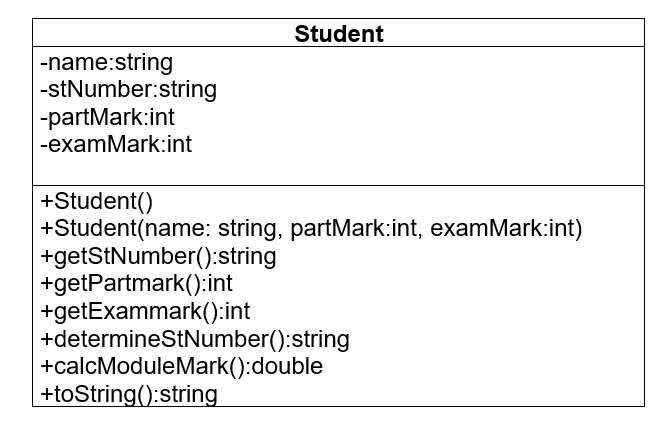
Extracted text: Student -name:string |-stNumber:string -partMark:int -examMark:int +Student() +Student(name: string, partMark:int, examMark:int) +getStNumber():string +getPartmark():int +getExammark():int +determineStNumber():string +calcModuleMark():double +toString():string