int main()
{
// declaring the character array
char word[100];
// taking user input of the word
printf("Enter the word: ");
scanf("%s", word);
// calling the hasVowel() function and passing the word with it
// storing the returned value in a variable result
int result = hasVowel(word);
// if the result returned is 1
if(result == 1)
{
printf("There is a vowel in the word \"%s\"", word);
}
// if the result returned is 0
else if(result == 0)
{
printf("There is no vowel in the word \"%s\"", word);
}
return 0;
}
2. it says that it is wrong but when I run the program the output is okay, I don't know what's wrong:
source code:
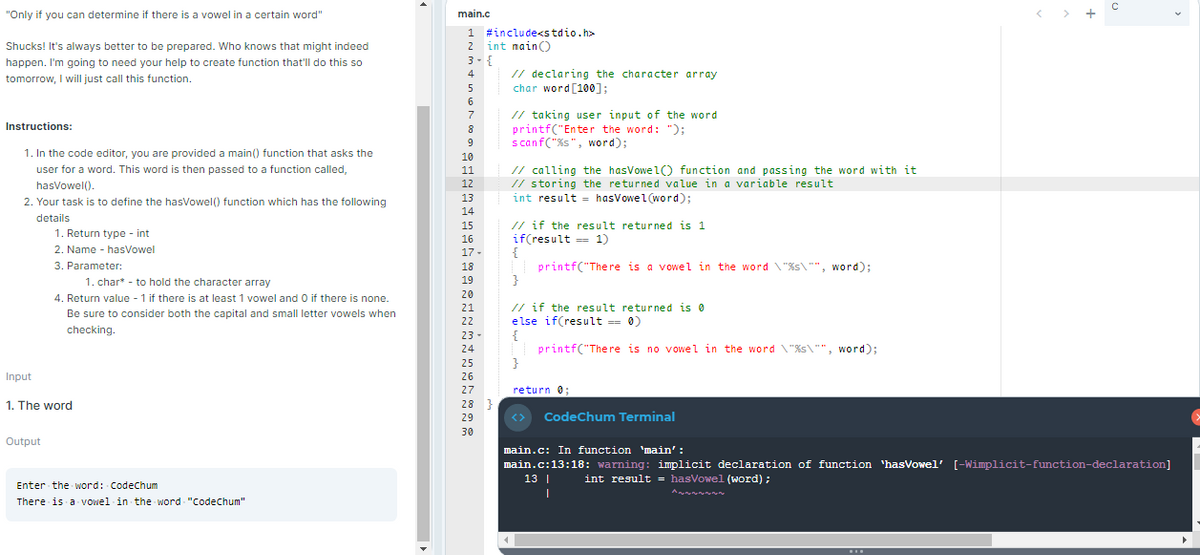
2 int nain) 3- { Shucks! It's always better to be prepared. Who knows that might indeed happen. I'm going to need your help to create function that'll do this so // declaring the character array char word [100]; 4 tomorrow, I will just call this function. 6 // taking user input of the word printf("Enter the word: "); scanf("%s", word); 7 Instructions: 8 9 1. In the code editor, you are provided a main() function that asks the 10 // calling the hasVowel) function and passing the word with it // storing the returned value in a variable result int result = hasVowel(word); user for a word. This word is then passed to a function called, 11 hasVowel(). 12 13 2. Your task is to define the hasVowel() function which has the following 14 details // if the result returned is 1 if(result == 1) { printf("There is a vowel in the word "%s\"", word); } 15 1. Return type - int 16 2. Name - hasVowel 17 - 3. Parameter: 18 1. char* - to hold the character array 19 20 4. Return value - 1 if there is at least 1 vowel and O if there is none. // if the result returned is 0 else if(result = 0) { printf("There is no vowel in the word \"%s\"", word); 21 Be sure to consider both the capital and small letter vowels when 22 checking. 23 - 24 25 Input 26 27 return 0; 1. The word 28 } 29 <> CodeChum Terminal 30 Output main.c: In function 'main' : main.c:13:18: warning: implicit declaration of function 'hasowel' [-Wimplicit-function-declaration] 13 | int result = hasVowel (word) ; Enter the word: Codechum There is a vowel in the word "Codechum" ... "/>
Extracted text: "Only if you can determine if there is a vowel in a certain word" main.c 1 #include 2 int nain) 3- { Shucks! It's always better to be prepared. Who knows that might indeed happen. I'm going to need your help to create function that'll do this so // declaring the character array char word [100]; 4 tomorrow, I will just call this function. 6 // taking user input of the word printf("Enter the word: "); scanf("%s", word); 7 Instructions: 8 9 1. In the code editor, you are provided a main() function that asks the 10 // calling the hasVowel) function and passing the word with it // storing the returned value in a variable result int result = hasVowel(word); user for a word. This word is then passed to a function called, 11 hasVowel(). 12 13 2. Your task is to define the hasVowel() function which has the following 14 details // if the result returned is 1 if(result == 1) { printf("There is a vowel in the word "%s\"", word); } 15 1. Return type - int 16 2. Name - hasVowel 17 - 3. Parameter: 18 1. char* - to hold the character array 19 20 4. Return value - 1 if there is at least 1 vowel and O if there is none. // if the result returned is 0 else if(result = 0) { printf("There is no vowel in the word \"%s\"", word); 21 Be sure to consider both the capital and small letter vowels when 22 checking. 23 - 24 25 Input 26 27 return 0; 1. The word 28 } 29 <> CodeChum Terminal 30 Output main.c: In function 'main' : main.c:13:18: warning: implicit declaration of function 'hasowel' [-Wimplicit-function-declaration] 13 | int result = hasVowel (word) ; Enter the word: Codechum There is a vowel in the word "Codechum" ...
![Can you help me find the ring that she wants?<br>< ><br>Test Cases<br>main.c<br>1 #include <stdio.h><br>int findRing (int rings 0, intn, int wantedRing)<br>3- {int index=0;<br>2<br>Instructions:<br>E Run Tests<br>for Çint i-0;i<n;i++)<br>{<br>if(rings [i]=wantedRing)//Performing a linear search to find the wanted ring<br>1. In the code editor, you are provided with the main() function that asks<br>4<br>5-<br>the user for 10 elements that represents the options for the ring as well<br>as a single integer value that represents the ring that she wants. Then,<br>a call to the findRing() function is made and the array of rings and the<br>6<br>Test Case 1<br>7-<br>8<br>index=i;<br>ring she wants are passed into it.<br>Your Output<br>}<br>}<br>9<br>2. Your task is to implement the findRing() function. This has the following<br>details:<br>10<br>return index;<br>Enter option #1:<br>Enter option #2:<br>11<br>1. Return type - int<br>2. Name - findRing<br>12 }<br>13 - int main) {<br>Enter option #3:<br>:<br>Enter option #4:<br>3. Parameters<br>14<br>int rings [10];<br>1. int* - for the array of rings<br>15<br>Enter option #5:<br>for Çint i = 0; i <10; i++) {<br>printf(](https://s3.us-east-1.amazonaws.com/storage.unifolks.com/qimg-008/008_a7kcxz0-e045i1v2.png)
Extracted text: Can you help me find the ring that she wants? <> Test Cases main.c 1 #include int findRing (int rings 0, intn, int wantedRing) 3- {int index=0; 2 Instructions: E Run Tests for Çint i-0;i<10; i++) { printf("enter option #%d: ", i + 1); scanf("%d", &rings [i]): } 16 - 2. int - size of the array of rings enter option #6: 17 3. int - the ring that she wants enter option #7: 18 4. return value - the index of the ring in the array of rings. it is guaranteed that there is only one such ring that matches the ring that she wants. enter option #s: 19 20 enter option #9: 21 enter option #10: int wantedring; printf("enter the ring she wants: "); scanf("%d", &wantedring); 22 enter the ring sh 23 input 24 ring 25 found at 25 printf("\nring %d found at option %d!", wantedring, findring (rings, 10, wantedring) + 1); 1. ten integer values representing the rings 26 27 return 0; 2. integer value representing the ring she wants 28 expected output 29 } output enter option #1: enter option #2: enter option #3: enter option #1: 5 enter option #4: enter option #5: enter option #2: 3 enter option #3: 10 enter enter option #6: enter option #4: 9 enter option #5: 13 enter option #7: enter option #6: 11 enter option #7: 20 enter option #8: enter option #9: enter option #10: i++)="" {="" printf("enter="" option="" #%d:="" ",="" i="" +="" 1);="" scanf("%d",="" &rings="" [i]):="" }="" 16="" -="" 2.="" int="" -="" size="" of="" the="" array="" of="" rings="" enter="" option="" #6:="" 17="" 3.="" int="" -="" the="" ring="" that="" she="" wants="" enter="" option="" #7:="" 18="" 4.="" return="" value="" -="" the="" index="" of="" the="" ring="" in="" the="" array="" of="" rings.="" it="" is="" guaranteed="" that="" there="" is="" only="" one="" such="" ring="" that="" matches="" the="" ring="" that="" she="" wants.="" enter="" option="" #s:="" 19="" 20="" enter="" option="" #9:="" 21="" enter="" option="" #10:="" int="" wantedring;="" printf("enter="" the="" ring="" she="" wants:="" ");="" scanf("%d",="" &wantedring);="" 22="" enter="" the="" ring="" sh="" 23="" input="" 24="" ring="" 25="" found="" at="" 25="" printf("\nring="" %d="" found="" at="" option="" %d!",="" wantedring,="" findring="" (rings,="" 10,="" wantedring)="" +="" 1);="" 1.="" ten="" integer="" values="" representing="" the="" rings="" 26="" 27="" return="" 0;="" 2.="" integer="" value="" representing="" the="" ring="" she="" wants="" 28="" expected="" output="" 29="" }="" output="" enter="" option="" #1:="" enter="" option="" #2:="" enter="" option="" #3:="" enter="" option="" #1:="" 5="" enter="" option="" #4:="" enter="" option="" #5:="" enter="" option="" #2:="" 3="" enter="" option="" #3:="" 10="" enter="" enter="" option="" #6:="" enter="" option="" #4:="" 9="" enter="" option="" #5:="" 13="" enter="" option="" #7:="" enter="" option="" #6:="" 11="" enter="" option="" #7:="" 20="" enter="" option="" #8:="" enter="" option="" #9:="" enter="" option="">10; i++) { printf("enter option #%d: ", i + 1); scanf("%d", &rings [i]): } 16 - 2. int - size of the array of rings enter option #6: 17 3. int - the ring that she wants enter option #7: 18 4. return value - the index of the ring in the array of rings. it is guaranteed that there is only one such ring that matches the ring that she wants. enter option #s: 19 20 enter option #9: 21 enter option #10: int wantedring; printf("enter the ring she wants: "); scanf("%d", &wantedring); 22 enter the ring sh 23 input 24 ring 25 found at 25 printf("\nring %d found at option %d!", wantedring, findring (rings, 10, wantedring) + 1); 1. ten integer values representing the rings 26 27 return 0; 2. integer value representing the ring she wants 28 expected output 29 } output enter option #1: enter option #2: enter option #3: enter option #1: 5 enter option #4: enter option #5: enter option #2: 3 enter option #3: 10 enter enter option #6: enter option #4: 9 enter option #5: 13 enter option #7: enter option #6: 11 enter option #7: 20 enter option #8: enter option #9: enter option #10:>